Advanced shipping
API class /AEB/CL_PA_PB_CARRIER_BF
To use the full API functionality of Carrier Cloud for SAP, you can use this class: /AEB/CL_PA_PB_CARRIER_BF
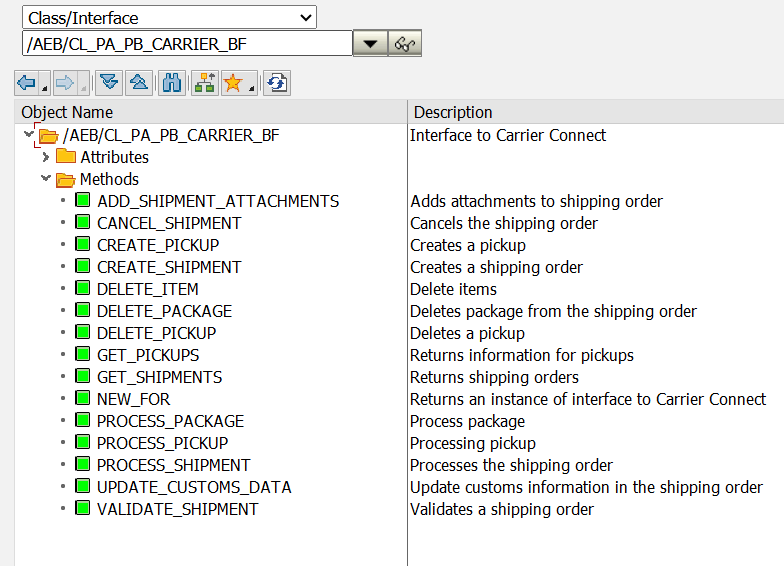
The API class provided by the add-on for SAP
This class enables you to call every available method and also change the field values and parameters completely flexible. For a detailed documentation of this API see here: API Documentation Carrier Cloud.
Supporting functions
Before you can use any of the methods from the class, e.g. Create_Shipment, certain mandatory data is needed. To support you gathering the required data, there are some complementary features, which will be explained in this section:
Task | Class |
---|---|
Determination of the org unit | TM document: /AE1/CL_PA_PB_FRO_OU_RULE_BC Delivery: /AEB/CL_PA_PB_DLV_OU_RULE_BC Shipment: /AEB/CL_PA_PB_SHP_OU_RULE_BC |
Determination of connection parms | /AEB/CL_PA_PB_ENGN_PRM_BC |
Collecting data from SAP business object ("Collector") This function provides the basic data based on the settings in the configuration. It runs also through the "AFTER_STD_FILLING"-method of the according BAdI for each object. | TM document: /AE1/CL_PA_PB_FRO_SHP_COLL_BC Delivery: /AEB/CL_PA_PB_DLV_SHP_COLL_BC Shipment: /AEB/CL_PA_PB_SHP_SHP_COLL_BC |
Determination of the workstation ID | /AEB/CL_PA_PB_WSTA_ID_BC |
Determination of the reference number | TM document: /AE1/CL_PA_PB_FRO_SHP_REF_BC Delivery: /AEB/CL_PA_PB_DLV_SHP_REF_BC Shipment: /AEB/CL_PA_PB_SHP_SHP_REF_BC |
The names of the classes are structured like this:
- /AEB/CL_PA_PB - Class is for public usage in customer implementations of Carrier Cloud for SAP
- DLV , SHP, FRO - the SAP document the class is applicable for: delivery, shipment or freight order (aka TM document)
- OU_RULE_BC, SHP_COLL_BC, SHP_REF_BC, WSTA_ID_BC - the functionality the class can be used for: organizational unit rule (OU_RULE), collect shipping data (SHIP_COLL), get reference (REF) or get workstation data (WSTA).
In this part of the guide we will walk through all those functions. All examples are based on an outbound delivery, but you can adapt them to other supported SAP business objects using the according class as listed above. Regardless of the SAP business object you're transferring, the result will be a "shipping order" in Carrier Cloud.
Organizational unit
First step is to determine the AEB specific organizational unit for a business object. The org unit is helpful to separate data access and read certain data from the configuration. This program determines the organizational unit for a delivery and writes it to the screen:
REPORT zaeb_create_first_shipment.
PARAMETERS: vbeln TYPE likp-vbeln.
DATA:
likp TYPE likp,
lipss TYPE STANDARD TABLE OF lips,
vbpas TYPE STANDARD TABLE OF vbpa,
vekps TYPE STANDARD TABLE OF vekp,
org_unit_rule_bc TYPE REF TO /aeb/cl_pa_pb_dlv_ou_rule_bc,
org_unit TYPE string.
SELECT SINGLE * FROM likp INTO likp WHERE vbeln = vbeln.
SELECT * FROM lips INTO TABLE lipss WHERE vbeln = vbeln.
SELECT * FROM vbpa INTO TABLE vbpas WHERE vbeln = vbeln.
SELECT * FROM vekp INTO TABLE vekps WHERE vpobjkey = vbeln.
org_unit_rule_bc = /aeb/cl_pa_pb_dlv_ou_rule_bc=>new_for(
im_likp = likp
im_lipss = lipss ).
org_unit = org_unit_rule_bc->get_org_unit( ).
WRITE: 'OrgUnit: ' , org_unit.
WRITE /.
Connection parameters
The next step is to read the connection parameters linked to this organizational unit. This connection is used to call the endpoint (the Carrier Cloud for SAP) eventually.
REPORT zaeb_create_first_shipment.
PARAMETERS: vbeln TYPE likp-vbeln.
DATA:
likp TYPE likp,
lipss TYPE STANDARD TABLE OF lips,
vbpas TYPE STANDARD TABLE OF vbpa,
vekps TYPE STANDARD TABLE OF vekp,
org_unit_rule_bc TYPE REF TO /aeb/cl_pa_pb_dlv_ou_rule_bc,
org_unit TYPE /aeb/01_char20,
engn_prm_bc TYPE REF TO /aeb/cl_pa_pb_engn_prm_bc,
engn_prm_mo TYPE REF TO /aeb/if_pa_pb_engn_prm_mo.
SELECT SINGLE * FROM likp INTO likp WHERE vbeln = vbeln.
SELECT * FROM lips INTO TABLE lipss WHERE vbeln = vbeln.
SELECT * FROM vbpa INTO TABLE vbpas WHERE vbeln = vbeln.
SELECT * FROM vekp INTO TABLE vekps WHERE vpobjkey = vbeln AND xyz -> select the right HUs here using further criteria...
*Org unit is required to read configured values
org_unit_rule_bc = /aeb/cl_pa_pb_dlv_ou_rule_bc=>new_for( im_likp = likp im_lipss = lipss ).
org_unit = org_unit_rule_bc->get_org_unit( ).
engn_prm_bc = /aeb/cl_pa_pb_engn_prm_bc=>get_instance( ).
engn_prm_mo = engn_prm_bc->get_engn_prm_do_for( org_unit ).
WRITE: 'Destination: ' , engn_prm_mo->get_destination( ).
WRITE: 'Engine client: ' , engn_prm_mo->get_engine_client( ).s
If you run the report again the destination and client for Carrier Cloud for SAP will be shown.
Collecting data from an SAP business object
Next, we need the data for the shipping order that we want to send to Carrier Cloud. Use the "collector"-class for the according SAP business object:
- Delivery: /AEB/CL_PA_PB_DLV_SHP_COLL_BC
- Shipment: /AEB/CL_PA_PB_SHP_SHP_COLL_BC
- TM document: /AE1/CL_PA_PB_FRO_SHP_COLL_BC
The collector provides the data based on the standard logic and configuration of the AEB add-on, plus the additional logic from the BAdIs that are implemented. Background: To change or add something for the collected data, you can implement different BAdIs for the according business object: BAdIs to change data .
Here is an example of how to collect the data for an outbound delivery:
REPORT zaeb_create_first_shipment.
PARAMETERS: vbeln TYPE likp-vbeln.
DATA:
likp TYPE likp,
lipss TYPE STANDARD TABLE OF lips,
vbpas TYPE STANDARD TABLE OF vbpa,
vekps TYPE STANDARD TABLE OF vekp,
org_unit_rule_bc TYPE REF TO /aeb/cl_pa_pb_dlv_ou_rule_bc,
org_unit TYPE /aeb/01_char20,
engn_prm_bc TYPE REF TO /aeb/cl_pa_pb_engn_prm_bc,
engn_prm_mo TYPE REF TO /aeb/if_pa_pb_engn_prm_mo,
collector_bc TYPE REF TO /aeb/cl_pa_pb_dlv_shp_coll_bc,
shipment TYPE /aeb/pa_pb_dl_shp_req_do.
SELECT SINGLE * FROM likp INTO likp WHERE vbeln = vbeln.
SELECT * FROM lips INTO TABLE lipss WHERE vbeln = vbeln.
SELECT * FROM vbpa INTO TABLE vbpas WHERE vbeln = vbeln.
SELECT * FROM vekp INTO TABLE vekps WHERE vpobjkey = vbeln AND xyz -> select the right HUs by using further criteria
org_unit_rule_bc = /aeb/cl_pa_pb_dlv_ou_rule_bc=>new_for( im_likp = likp im_lipss = lipss ).
org_unit = org_unit_rule_bc->get_org_unit( ).
collector_bc = /aeb/cl_pa_pb_dlv_shp_coll_bc=>new_for( im_likp = likp
im_lipss = lipss
im_vbpas = vbpas
im_vekps = vekps ).
shipment = collector_bc->create_shipment( org_unit ).
write shipment-referencenumber1.
WRITE /.
With this example you have collected the shipping data of the SAP delivery, e.g. the ship-to address and the carrier.
To change or add something for the collected data, you can implement the "AFTER_STD_FILLING"-method of the BAdI for the according business object: BAdIs to change data .
Determine the workstation
The workstation ID is mandatory for creating a shipping order and printing labels. Just add the following code lines to the program:
DATA: workstation_id TYPE string.
workstation_id = /aeb/cl_pa_pb_wsta_id_bc=>new( )->get_workstation_id( ).
write: workstation_id.
write /.
Determine the reference number
In certain scenarios & calls the reference number of the shipping order is required. During the creation of a shipping order in Carrier Connect a unique reference is assigned. By using this function, you can retrieve that reference ID for the according SAP business object:
REPORT zaeb_create_first_shipment.
PARAMETERS: vbeln TYPE likp-vbeln.
DATA:
likp TYPE likp,
lo_dlv_shp_ref TYPE REF TO /aeb/cl_pa_pb_dlv_shp_ref_bc,
ls_shp_ref TYPE /aeb/pa_pb_dl_shp_ref_do.
SELECT SINGLE * FROM likp INTO likp WHERE vbeln = vbeln.
lo_dlv_shp_ref = /aeb/cl_pa_pb_dlv_shp_ref_bc=>new_for( im_likp = likp ).
ls_shp_ref = lo_dlv_shp_ref->get_shp_ref_do( ).
write: ls_shp_ref.
write /.
Further subsequent functionality
Using the API class you can make use of further functionality:
Create shipping order (Create shipment)
Upddate shipping order (Process Shipment)
Read shipping order data (Get Shipments)
Updated 2 months ago